Singleton design pattern falls under creational design pattern category. It is one of the most argued design patterns in technical discussions.
This pattern ensures that only one instance of the class gets created at any point of time. It provides a global access method to instantiate or get the singleton object. The singleton object can be created by eager initialization or by lazy initialization.
Eager Initialization:
Creates the instance of single tone object during the class loading
Lazy Initialization:
Creates the single tone object when the global static method is called first time
Implementations:
- Define a private constructor so that it can’t be instantiated from other classes.
- Define a static variable to ensure that only one instance of the singleton object exist.
- Define a global static method which would ensure that either to create new object or to return the existing object.
- Make sure that only one thread can access the singleton class by making methods as synchronized
Advantages:
- This design patten is very simple and easy to implement
- This pattern is used in logging, catching and thread pool design etc.
- This pattern could improve the performance if the object is costly to create every time.
Note:
Use this pattern cautiously in multithreaded environment .
Example:
Consider a scenario where, a satellite communicating with multiple ground stations. Only one satellite object is allowed. If there is no satellite object then the global static method will create a satellite object. Otherwise, it will return the existing object.
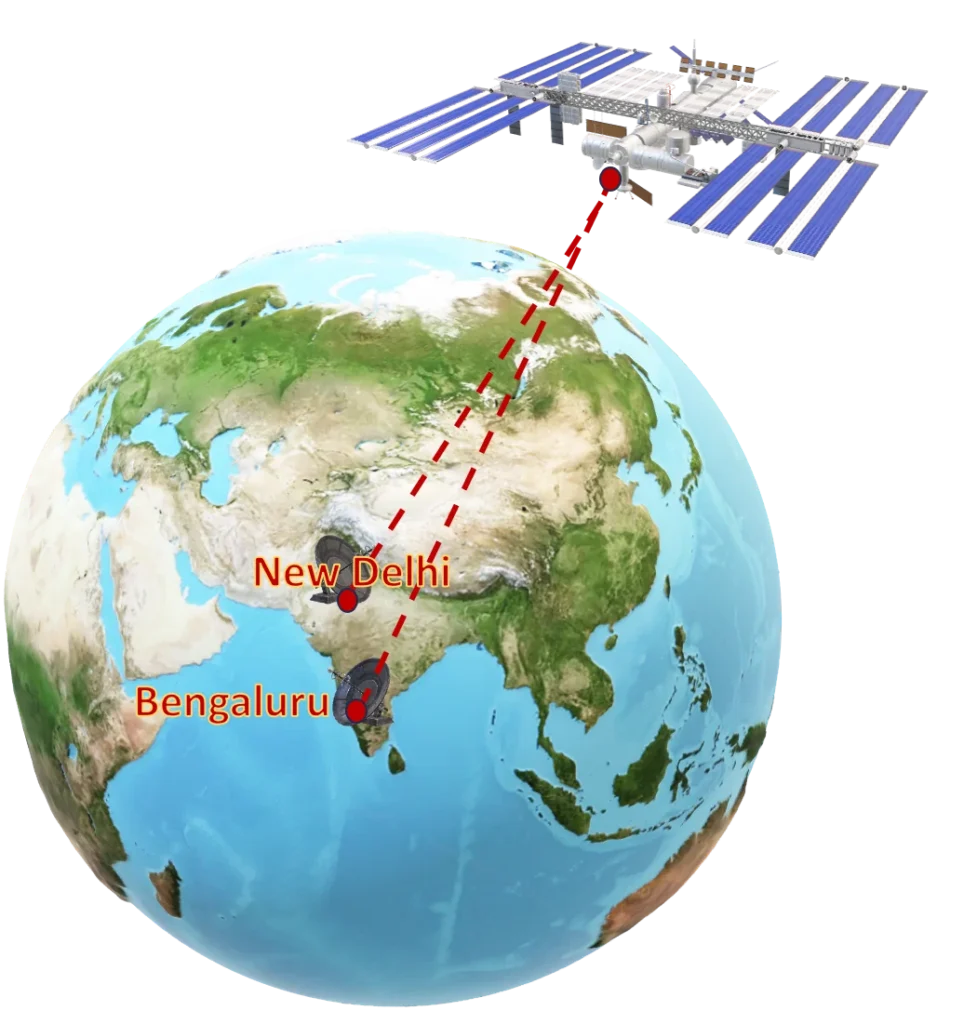
Design Diagram
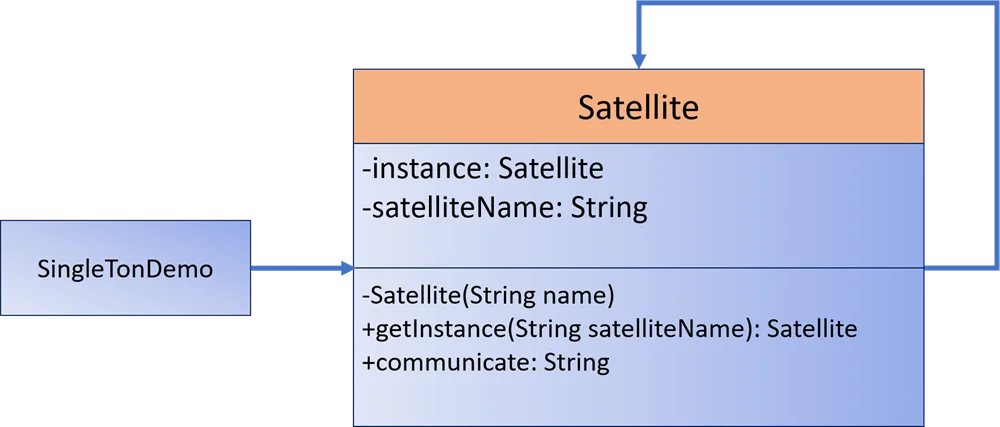
Creating Singleton Class
Here the satellite class is a singleton class, which restrict us to create only one satellite instance.
- Create a private constructor to restrict satellite object creation directly from outside the class.
- Create a static variable to ensure that only one satellite object exist.
- Create a global static synchronized method which would ensure that either to create new satellite object or to return the existing satellite object.
package com.spsn.learn.designpattern.singleton;
final public class Satellite {
private static Satellite instance;
private final String satelliteName;
private Satellite(String name) {
this.satelliteName = name;
System.out.println("Satellite Name : "+name+" created");
}
public static synchronized Satellite getInstance(String satelliteName) {
if (instance == null) {
instance = new Satellite(satelliteName);
}
else
{
System.out.println("Satellite Name :"+instance.satelliteName+" already exists, thus cannot create new satellite instance "+satelliteName);
}
return instance;
}
public String communicate() {
return " established communication with " + satelliteName;
}
}
Creating SatelliteGroundStation Class
SatelliteGroundstation class is a Runnable class which calls the global static method of the singleton class (Satellite class).
package com.spsn.learn.designpattern.singleton;
public class SatelliteGroundStation implements Runnable{
private String usage;
private String location;
private Satellite controlRoom;
public SatelliteGroundStation(String usage, String location, String satelliteName)
{
this.usage = usage;
this.location = location;
this.controlRoom = Satellite.getInstance(satelliteName);
}
@Override
public void run() {
System.out.println(usage+" ground station from "+ location +" has "+controlRoom.communicate());
}
}
Creating SingleTonDemo class
SingleTonDemo class is the main class which creates instances for the runnable class (SatelliteGroundstation).
package com.spsn.learn.designpattern.singleton;
public class SingleTonDemo {
public static void main(String[] args) {
SatelliteGroundStation bangalore = new SatelliteGroundStation("DeepSea-Exploration", "Bangalore" ,"INSAT");
SatelliteGroundStation delhi = new SatelliteGroundStation("Mining-Exploration", "Delhi","CHADRAYAN");
Thread bangaloreTh = new Thread(bangalore);
Thread delhiTh = new Thread(delhi);
bangaloreTh.start();
delhiTh.start();
}
}
Result
Satellite Name : INSAT created
Satellite Name :INSAT already exists, thus cannot create new satellite instance CHADRAYAN
DeepSea-Exploration ground station from Bangalore has established communication with INSAT
Mining-Exploration ground station from Delhi has established communication with INSAT